You might want to git pull all repos you cloned in a specific folder before starting to work on these projects.
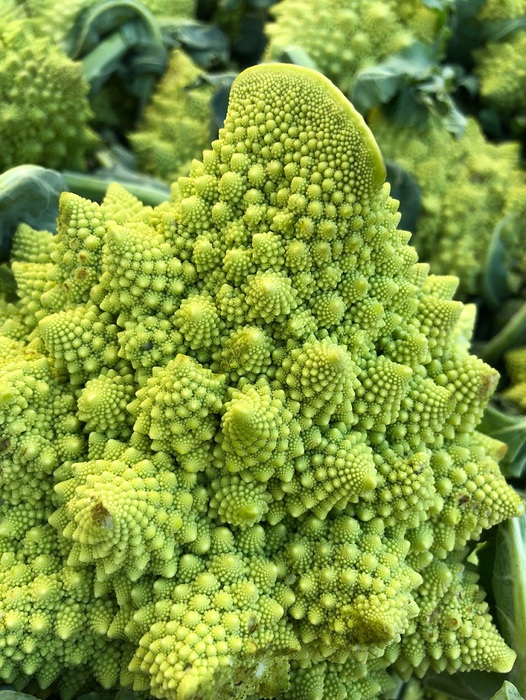
This is a simple script in both python and bash.
#!/usr/bin/python3
import os,sys
import git
from termcolor import colored
from argparse import ArgumentParser
def main(argv):
parser = ArgumentParser()
parser.add_argument("-d", dest="basedir", help="base directory", default='./')
args = parser.parse_args()
for root, dirs, files in os.walk(args.basedir):
for repo in dirs:
if repo.endswith(".git"):
pullresult = git.Repo(os.path.join(root, repo).replace(".git","")).remotes.origin.pull('master')
print(colored(str(os.path.join(root, repo).replace(".git","")), "green") + "\n" + str(pullresult))
if __name__ == "__main__":
main(sys.argv[1:])
#!/bin/bash
find . -name ".git" | awk 'BEGIN{FS=OFS="."}{NF--; print}' | xargs -I{} git -C {} pull