A simple script to locally clone a list of repos and bundle them. Will skip empty repos and bundle wikis as well. One list file in the same path is required, one repo per line in the format: https://git:MYGITTOKEN@git.server.address/path/repo.git
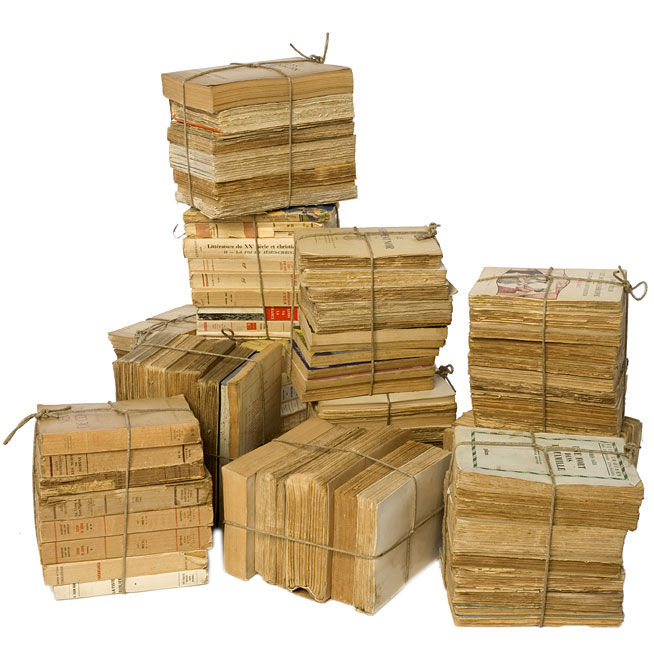
# Directory that hosts bundles
mkdir bundles
# Bundle all non-empty repos
while read line; do
git clone --mirror $line folder
cd folder
filename=$( echo $line | awk -F/ '{print $NF}')
# is repo empty?
if $( test -n "$(git rev-list -n1 --all 2>&1 2> /dev/null)" ) ; then
echo "Git repo has commits, bundling.."
git bundle create ../bundles/$filename.bundle --all
else
echo "Git repo has no commits, skipping"
fi
cd ..
rm -rf folder
done < list
# Bundle all non-empty wikis (we might as well have empty repos with wikis)
while read line; do
wikiurl=$( echo $line | sed 's/\.git/\.wiki\.git/g' )
git clone --mirror $wikiurl folder
cd folder
filename=$( echo $line | awk -F/ '{print $NF}')
# is repo empty?
if $( test -n "$(git rev-list -n1 --all 2>&1 2> /dev/null)" ) ; then
echo "Git repo has commits, bundling.."
git bundle create ../bundles/$filename.wiki.bundle --all
else
echo "Git repo has no commits, skipping"
fi
cd ..
rm -rf folder
done < list